Simple Todo App using Google Polymer
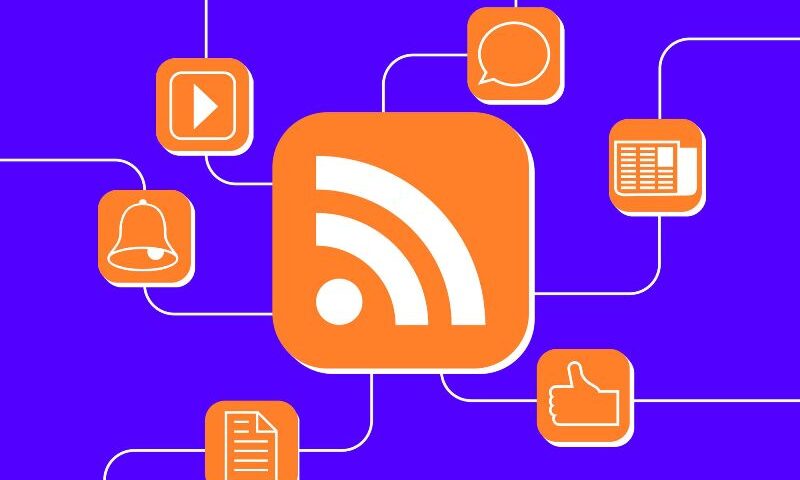
Polymer is a framework used to build Modern Mobile First Web Apps. If you are familiar with AngularJS, where we use Directives to create re-usable Components, similar way in Polymer we can extend the HTML and create custom Tag with a specific feature.
HTML5 has already introduced many Semantic Tags to make the html code more meaningful. Polymer takes a step further and makes it even structured and organised making HTML more simpler and maintainable.
WEB Components:
Polymer has basically two types of components:
- Core Components
- Material Components (Paper Components)
All core components include from design elements to the utility. Paper components include all the Material aspects like Buttons, Floating buttons, Header, Menus etc.
Example to make a simple AJAX call in Polymer:
We need to use a Core AJAX element to make a HTTP request:
1
2
3
4
5
6
|
<core–ajax id=“ajax”
auto
url=“http://URL_to_resource”
on–core–response=“{{reponseHandler}}”
handleAs=“json”>
</core–ajax>
|
Creating a custom Element:
Using <polymer-element> we can create a custom Element. Also we can import any other elements using <link rel=”import”>.
And every Element contains basically two parts:
- <Template> : provide the html to your Element to be replaced. (can additionally have a <style> to the template)
- <Script> : Add functionality or interaction to the Element.
Here is an Example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
<!— Imports —>
<link rel=“import” href=“../../bower_components/polymer/polymer.html”>
<link rel=“import” href=“../../bower_components/core-icon-button/core-icon-button.html”>
<!— Polymer Element —>
<polymer–element name=“element-name” attributes=“any-inputs”>
<template>
<link rel=“stylesheet” href=“any-style.css”>
<!— Content of the Element —>
<content select=“core-label”></content>
</template>
<script>
(function() {
Polymer({
// Polymer is ready:
});
})();
</script>
</polymer–element>
|
Polymer + Material Design:
Polymer comes with Many Material components pre included. Check out the link for some basic components: https://polymer-library.polymer-project.org/3.0/docs/devguide/custom-elements
Polymer Todo:
Lets build a Simple Todo app using Polymer. Whole purpose is to build a fully responsive App to make it work on Mobile as well on WEB (A single Code Base :)). These are the features covered:
- Listing Todos
- Add a new Todo
- Mark as completed
- Remove completed
- Clear All
Screenshots:


Basic setup:
Using a Yeoman Generator: https://github.com/yeoman/generator-polymer
YOLOmer! Polymer and Yeoman for lighting fast dev, by Rob Dodson:
1
|
<script src=“bower_components/webcomponentsjs/webcomponents.js”></script>
|
A self explained source code is here at GIT: https://github.com/chanusukarno/Polymer-Todo